XamDataGrid の Field に ViewModel のデータをバインドする方法は以前別の記事(XamDataGrid Field に ViewModel のデータをバインドする)でご紹介しておりますが、今回はコードビハインドでバインドする方法をご紹介致します。
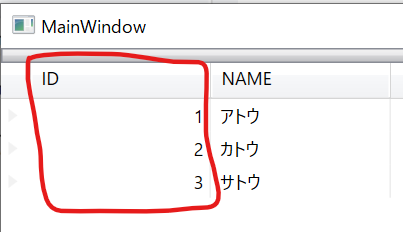
今回はこちらの画像のようなレイアウトに対し、ID 列を編集不可とするため、 Field の AllowEdit プロパティ に false を設定したいと思います。
MainWindow.xaml では XamDataGrid を用意する
Xaml では XamDataGrid の定義のみ行います。
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:XamDataGrid_sample" x:Class="XamDataGrid_sample.MainWindow" xmlns:igDP="http://infragistics.com/DataPresenter" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800" Loaded="Window_Loaded"> <Window.DataContext> <local:MainWindowViewModel/> </Window.DataContext> <Grid> <!-- XamDataGrid を定義 --> <igDP:XamDataGrid x:Name="xamDataGrid1"> </igDP:XamDataGrid> </Grid> </Window>
MainWindowViewModel.cs でプロパティを用意する
ViewModel では、編集可否を制御するフラグ、IsAllowEdit プロパティ(デフォルト値 false)を用意します。
internal class MainWindowViewModel : NotificationObject { private ObservableCollection<Item> _items; public ObservableCollection<Item> Items { get { return _items; } set { _items = value; OnPropertyChanged(); } } // ★IsAllowEdit を用意 private bool _isAllowEdit; public bool IsAllowEdit { get { return _isAllowEdit; } set { _isAllowEdit = value; OnPropertyChanged(); } } public MainWindowViewModel() { _items = new ObservableCollection<Item>(); _items.Add(new Item { Id = 1, Name = "アトウ" }); _items.Add(new Item { Id = 2, Name = "カトウ" }); _items.Add(new Item { Id = 3, Name = "サトウ" }); // ★Id 列を編集不可とするため、_isAllowEdit に false を設定しておく _isAllowEdit = false; } }
MainWindow.xaml.cs でバインドの処理を実装する
コードビハインドでは ID 列のオブジェクトを生成し、ViewModel で用意した IsAllowEdit をバインドする処理を実装します。
SetBinding では、バインディングのターゲットプロパティに「Field.AllowEditProperty」を設定します。
public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void Window_Loaded(object sender, RoutedEventArgs e) { xamDataGrid1.FieldLayoutSettings.AutoGenerateFields = false; FieldLayout fieldLayout1 = new FieldLayout(); fieldLayout1.Key = "FieldLayout1"; xamDataGrid1.FieldLayouts.Add(fieldLayout1); // ★Id 列を編集不可とするため、Id列のFiledオブジェクトを生成 var idField = new Field("Id"); idField.Name = "Id"; idField.Label = "ID"; // ★Id 列の IsAllowEdit(初期値が false)をBinding する var binding = new Binding { Path = new PropertyPath("DataContext.IsAllowEdit"), RelativeSource = RelativeSource.Self }; // ★ Field.AllowEditProperty にバインド BindingOperations.SetBinding(idField, Field.AllowEditProperty, binding); // ★idField を追加 fieldLayout1.Fields.Add(idField); var nameField = new Field("Name"); nameField.Name = "Name"; nameField.Label = "NAME"; fieldLayout1.Fields.Add(nameField); var dataSourceBinding = new Binding { Path = new PropertyPath("Items") }; BindingOperations.SetBinding(xamDataGrid1, XamDataGrid.DataSourceProperty, dataSourceBinding); } }
以上の方法で、XamDataGrid の Field にコードビハインドでのバインドを実装することができます。
サンプルもございますので、お試しください。