UltraDockManager でペインをクローズできないようにする場合は、
- 「閉じる」ボタンを消す。
- コンテキスト メニューの「非表示」を削除する。
をします。
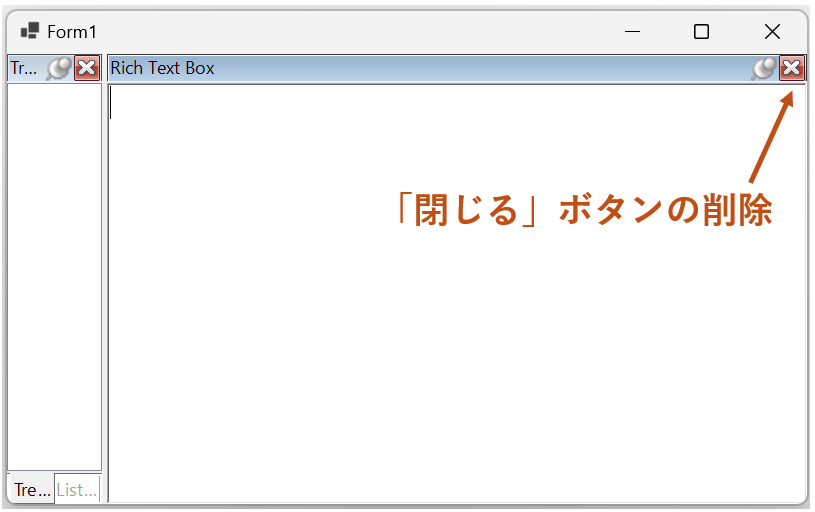
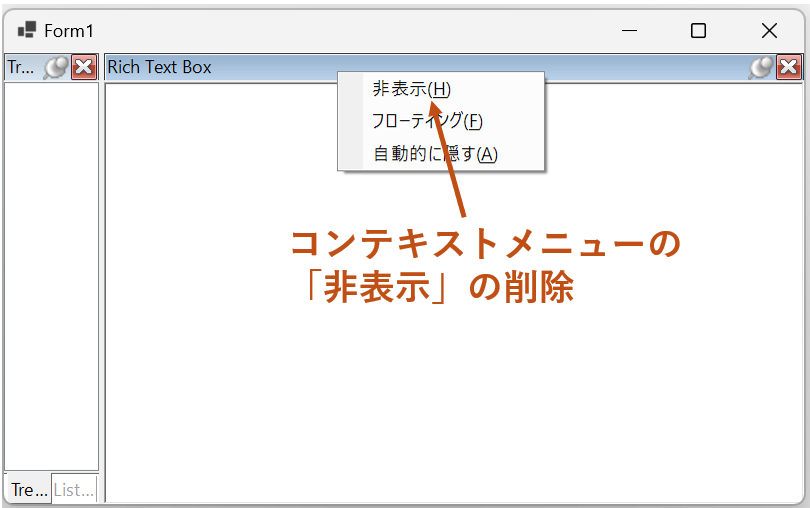
では、上のスクリーンショットの「Rich Text Box」のペインを使って実装していきましょう。
「閉じる」ボタンを消す
「Rich Text Box」の DockableControlPane の AllowClose プロパティに False を指定して「閉じる」ボタンを無効化します。このままではグレー アウトされた状態で表示され続けてしまうので、さらに ultraDockManager の ShowDisabledButtons を false に設定します。これで無効化されたボタンが消えます。
// dcpRichText は「Rich Text Box」の DockableControlPane ペインという想定です。 dcpRichText.Settings.AllowClose = Infragistics.Win.DefaultableBoolean.False; ultraDockManager1.ShowDisabledButtons = false;
コンテキストメニューの「非表示」を削除する
コンテキスト メニューからの「非表示」の削除はイベント ハンドラーで実装します。
まずマウス カーソルがペインのキャプション領域に入っている間だけ MouseDown イベントのイベント ハンドラーをアタッチします。
private void ultraDockManager1_MouseEnterElement(object sender, Infragistics.Win.UIElementEventArgs e) { var paneCaptionUIElement = e.Element as PaneCaptionUIElement; if (paneCaptionUIElement == null) return; paneCaptionUIElement.Control.MouseDown += OnMouseDown; } private void ultraDockManager1_MouseLeaveElement(object sender, Infragistics.Win.UIElementEventArgs e) { var paneCaptionUIElement = e.Element as PaneCaptionUIElement; if (paneCaptionUIElement == null) return; paneCaptionUIElement.Control.MouseDown -= OnMouseDown; }
次にアタッチした MouseDown イベント ハンドラーで ContextMenuStrip の Opening イベントを拾います。
private bool _isContextMenuStripOpeningSubscribed = false; private void OnMouseDown(object? sender, MouseEventArgs e) { var dockableWindow = sender as DockableWindow; if (_isContextMenuStripOpeningSubscribed || dockableWindow == null) return; var contextMenuStrip = dockableWindow.ContextMenuStrip; if (contextMenuStrip == null) return; contextMenuStrip.Opening += ContextMenuStrip_Opening; _isContextMenuStripOpeningSubscribed = true; }
※註: この記事では .NET 8 の前提で執筆しています。.NET Framework の場合は ContextMenuStripではなく、ContextMenu の Popup イベントを使用してください。
// .NET Framework の場合 var contextMenu = ((Control)sender).ContextMenu; contextMenu.Popup += ContextMenu_Popup;
最後に ContextMenuStrip の Opening イベント ハンドラーで、「Rich Text Box」のペインのコンテキスト メニューから「非表示」を削除します。
private void ContextMenuStrip_Opening(object? sender, System.ComponentModel.CancelEventArgs e) { var contextMenuStrip = sender as ContextMenuStrip; if(contextMenuStrip == null) return; ToolStripItem[] toolStripItems = new ToolStripItem[contextMenuStrip.Items.Count]; contextMenuStrip.Items.CopyTo(toolStripItems, 0); var hideItem = toolStripItems.ToList().FirstOrDefault(menuItem => menuItem?.Text?.Contains("非表示") ?? false); // "rtb" は「Rich Text Box」のペインの Key という想定です。 if ((contextMenuStrip.SourceControl as DockableWindow)?.Pane.Key == "rtb" && hideItem != null) { contextMenuStrip.Items.Remove(hideItem); } }
※註: この記事では .NET 8 の前提で執筆しています。.NET Framework の場合は以下の通りです。
// .NET Framework の場合 private void ContextMenu_Popup(object sender, EventArgs e) { var igContextMenu = (Infragistics.Win.IGControls.IGContextMenu)sender; var hideItem = igContextMenu.MenuItems.OfType<MenuItem>().FirstOrDefault(menuItem => menuItem.Text.Contains("非表示")); if ((igContextMenu.SourceControl as DockableWindow)?.Pane.Key == "rtb" && hideItem != null) { igContextMenu.MenuItems.Remove(hideItem); } }
以上で完了です!
実行結果
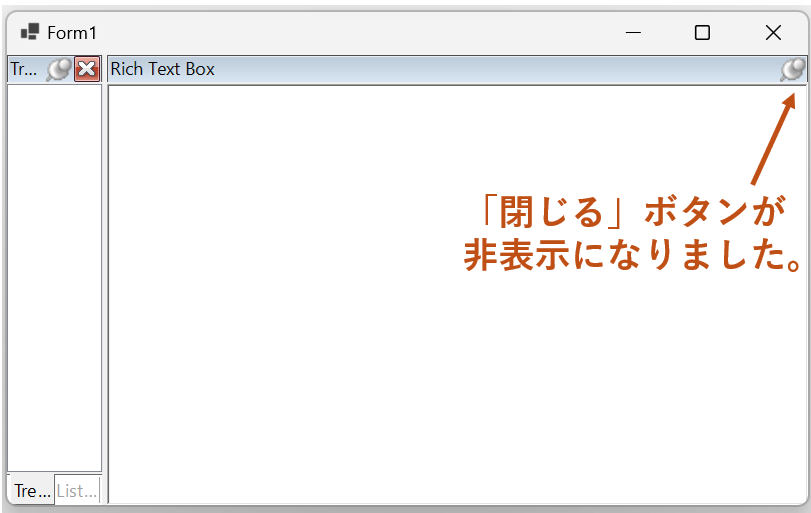
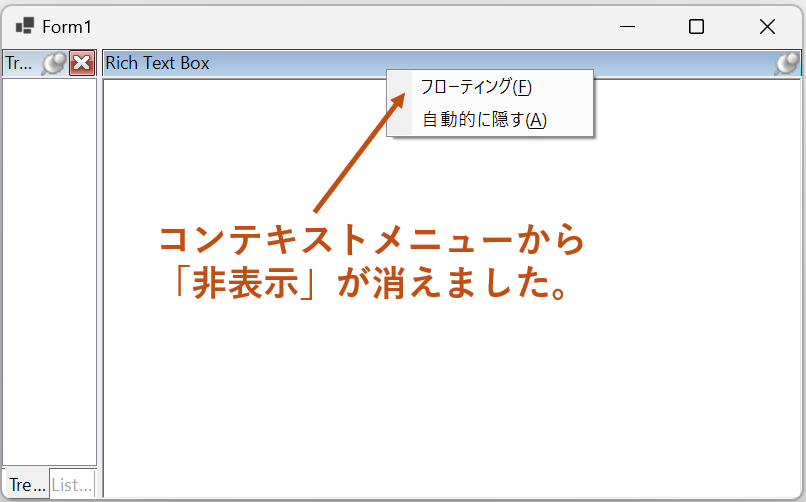